When writing tests, I often find myself switching between the IDE and terminal windows to actually run the tests. On projects with very large test suites this would often lead to situations where I’d spend time waiting for all the tests to finish.
I’d get distracted with something else and lose my focus or come back and find out one failed in the end. The best way to avoid this is to keep your feedback loops short.
There are some ways to improve this experience such as using PHPUnit groups or the –filter option but even those require some setup and switching between windows (or having to google the syntax on how to run just one test method in a file).
One day I was pairing with someone who used Visual Studio Code and I was amazed to see how they were able to run a single test method from within their editor by simply clicking a button. They would even get instant feedback visible within their editor. I thought I was dreaming and kept wondering if PHPStorm had a similar feature that I had just never realized.
It turns out that it does and it’s actually pretty easy to set up!
Getting started
I’m going to assume that you already have PHPUnit configured and are able to run it in another way.The first thing that we’ll do is make PHPStorm aware of our PHPUnit installation. This should in most cases already be configured automatically. If you’re unsure, you can verify in your preferences under PHP > Test Frameworks. You should see something like this:
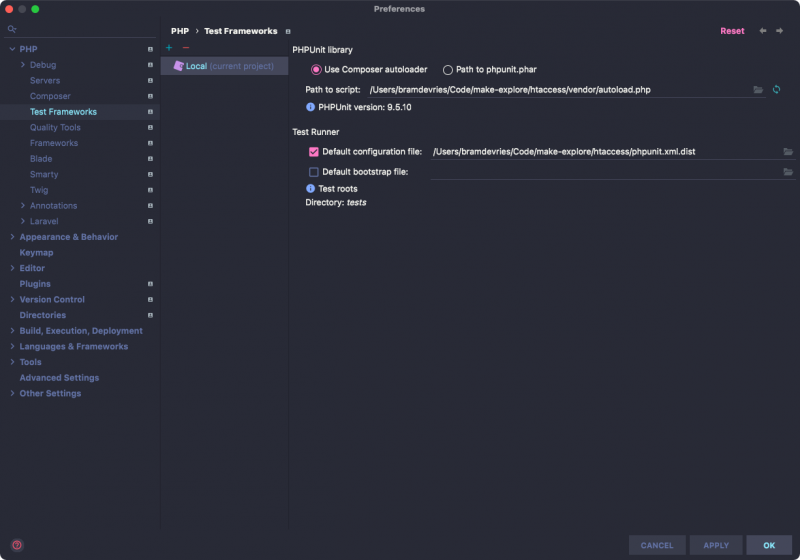
If you don’t see anything you can create a new entry by choosing PHPUnit Local and filling out the configuration.
Once that’s done we can do a first run of all of our tests by going to Run > Run…. This will prompt you with a dialog to further configure your test runner.
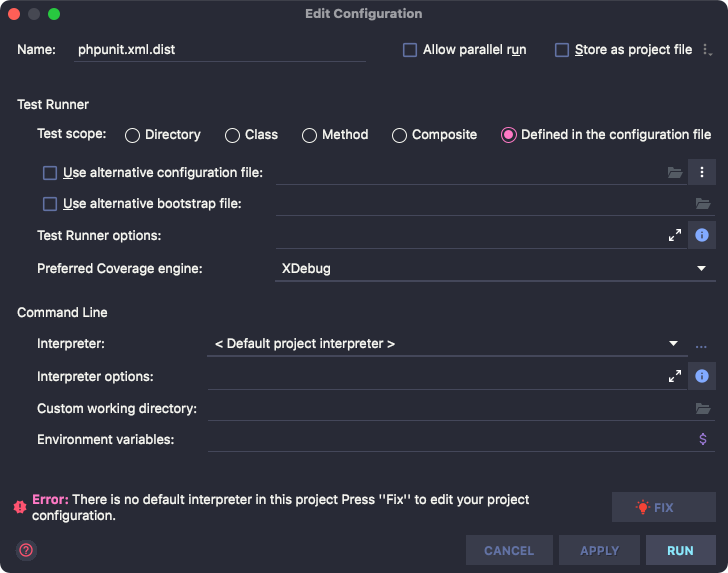
In the above screenshot you can see that there is an error that popped up because I don’t have an interpreter configured for the CLI. Thankfully PHPStorm offers me a “Fix” button that will guide me to a solution:
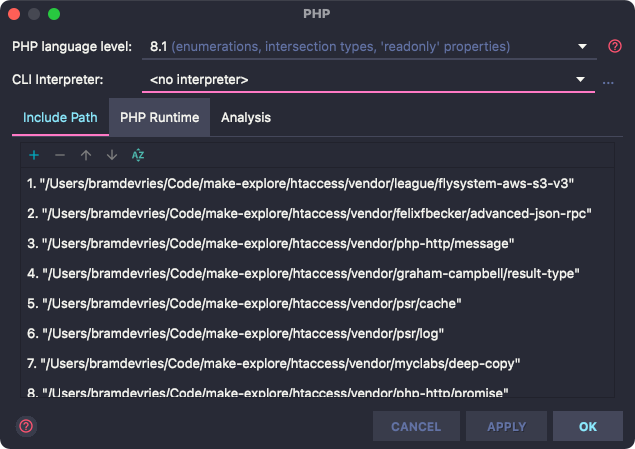
Interpreters are actually just the different PHP versions that PHPStorm is aware of. In this case the error occurs because the project is using PHP 8.1 which I do not have installed locally. So in this case I will first have to install it locally to avoid compatibility issues with the codebase.
Once that’s done I can continue with running the tests. This will open up the test runner tool and you will see the output of your test run:
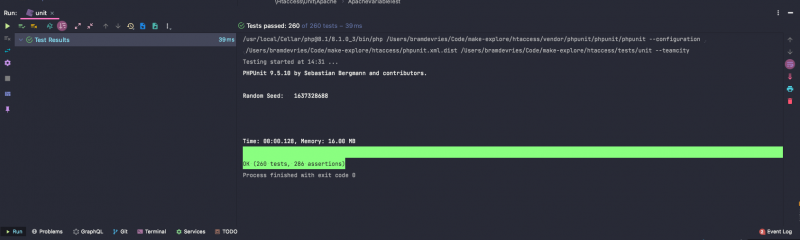
The cool thing is that we can do the same for individual tests:
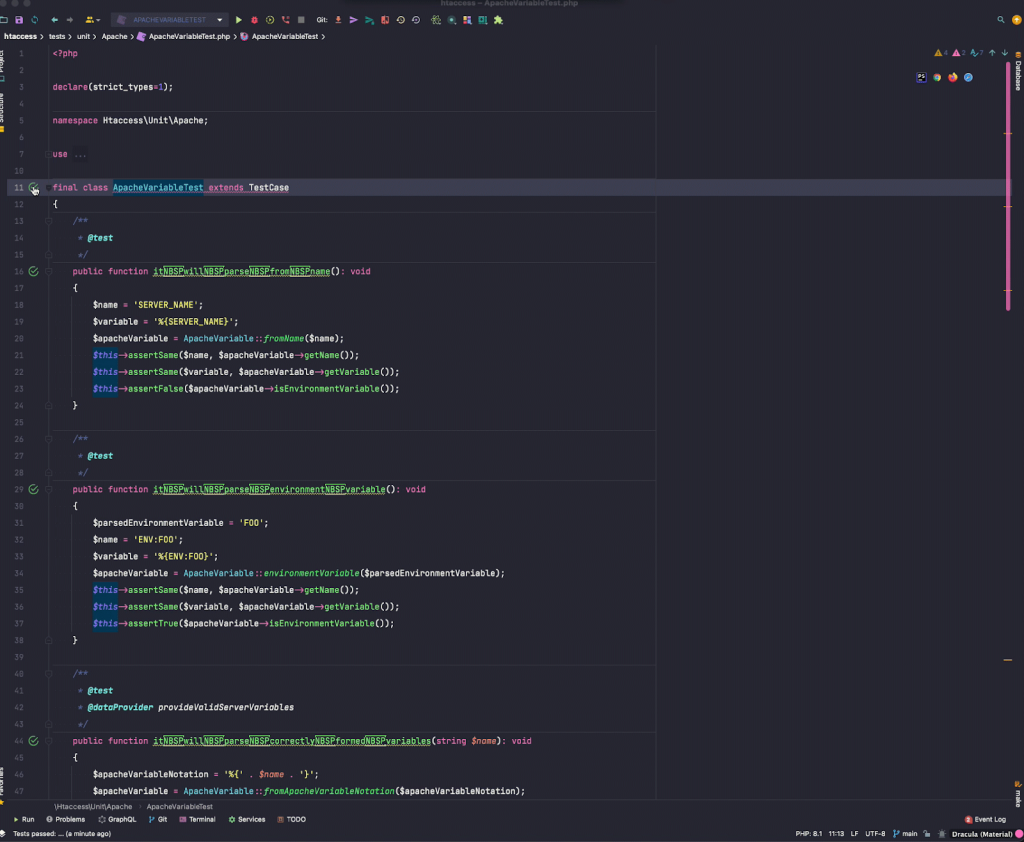
What about code coverage?
It can be useful to want to know the coverage of a certain test. This is also something that’s possible with PHPStorm by using the Run > Run “X” with Coverage option. This does require you to also install a coverage driver for the PHP version you are using. Personally, I like using pcov as it’s much faster than Xdebug.
Running with coverage is very similar to a regular test run. The only difference is that after the tests are successful you will see an overview of the coverage and it even shows inline if a certain part is not covered by a test:
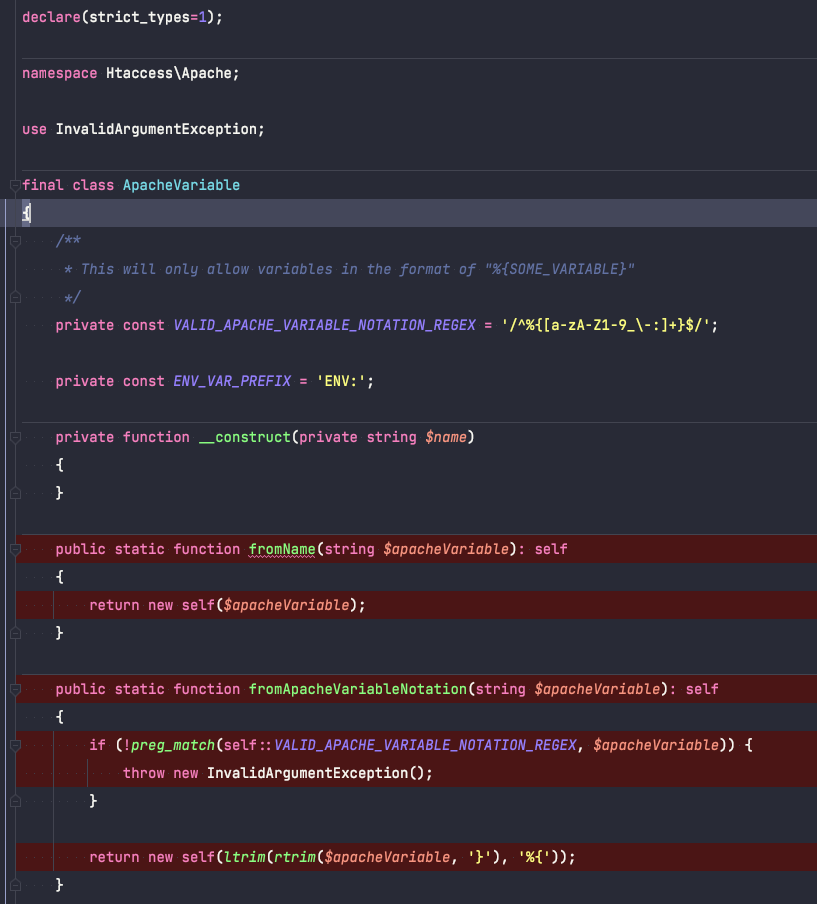
How about Docker?
So far we’ve been relying on locally installed PHP versions. For projects that include a Docker-based environment, we can leverage that when running our tests. In order to do so we’ll need to go back and add an additional test framework in our preferences under PHP > Test Frameworks.
Instead, we’ll now choose PHPUnit by Remote Interpreter.
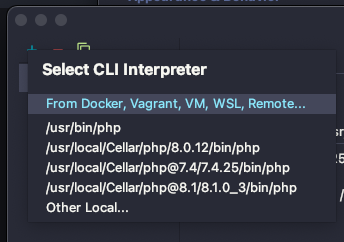
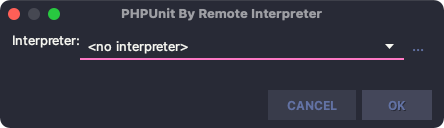
This will prompt you to choose an interpreter. It’s likely that the list you see is empty so we’ll have to create a new one first by choosing the From Docker, Vagrant, VM, WSL, Remote… option.
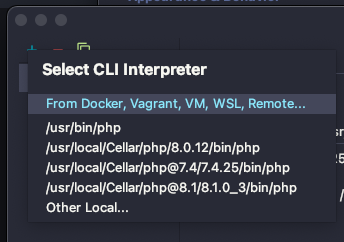
This opens up yet another dialog where you can choose the type of remote connection to use. In my case I’m using Docker Compose. This gives me the option to choose which service is running PHP based on the docker-compose.yml file that is included.
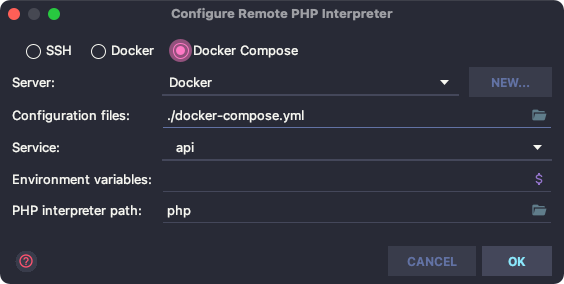
Once done, the interpreter should appear in the list from before, alongside some Docker Compose specific options.
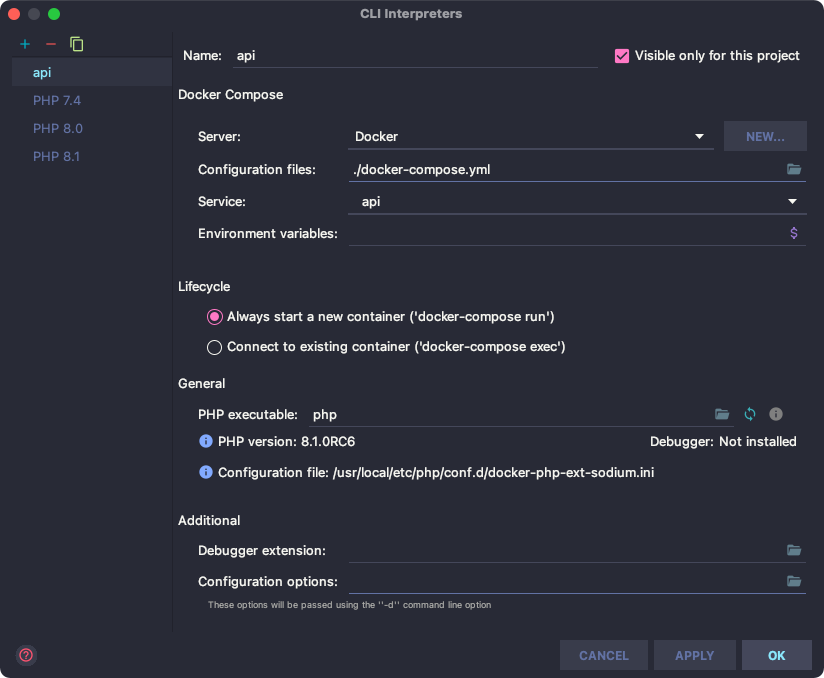
Now we can choose this interpreter for our test framework. Again, a lot of this will be configured automatically for you. To verify, make sure that a PHPUnit version is being displayed.
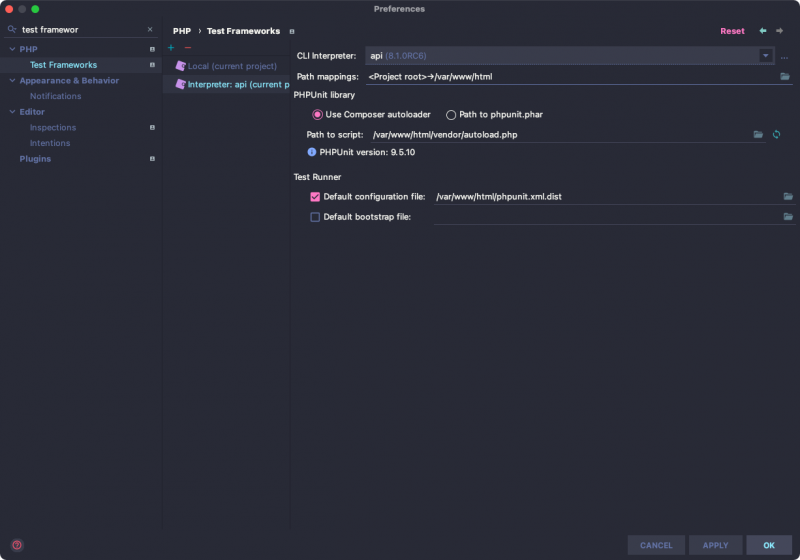
Now, as the final step, we can configure our default CLI interpreter to be the one that we just created. Now, our tests should run through docker-compose instead of our local PHP version:
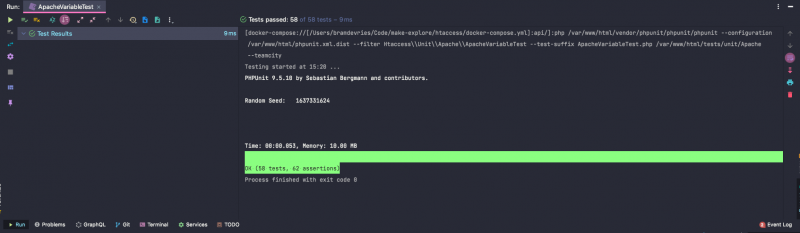
To conclude
For me, the ability to run tests with the click of a button and keeping the feedback loop short while I’m writing them has been a massive boost in productivity.
While this only talks about PHPUnit you can do the same for other test runners like Jest or Cypress and have a similar workflow.
Member discussion